
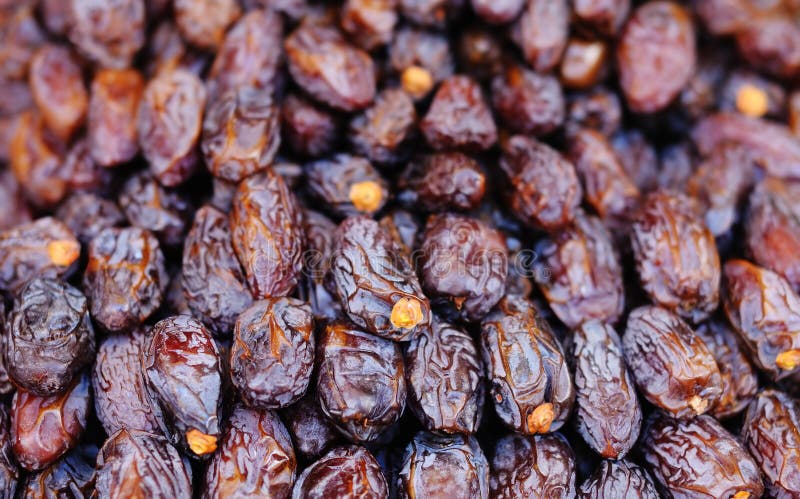
from bigtree import Node, find_children, find_child, find_child_by_name root = Node ( "a", age = 90 ) b = Node ( "b", age = 65, parent = root ) c = Node ( "c", age = 60, parent = root ) d = Node ( "c", age = 40, parent = c ) root. This does not require traversing the whole tree to find the node(s). It is also possible to search for one or more child node(s) based on attributes, and the search will be faster as Or using bitshift operator with the convention parent_node > child_node or child_node > b root > c d = 65 ) # (Node(/a, age=90), Node(/a/b, age=65)) find_names ( root, "c" ) # (Node(/a/c, age=60), Node(/a/c/c, age=40)) find_paths ( root, "/c" ) # partial path # (Node(/a/c, age=60), Node(/a/c/c, age=40)) find_attrs ( root, "age", 40 ) # (Node(/a/c/c, age=40),) Nodes can be linked to each other with parent and children setter methods, Nodes can have attributes if they are initialized from Node, dictionary, or pandas DataFrame. Run the following lines in command prompt: $ pip install 'bigtree' If tree needs to be exported to image, it requires additional dependencies. To install bigtree, run the following line in command prompt: $ pip install bigtree
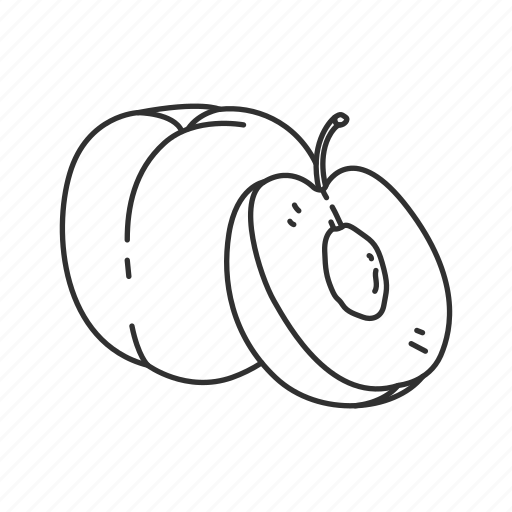
Export to list, dictionary, or pandas DataFrame.From list, containing parent-child tuples.DAGNode, extendable class for constructing Directed Acyclic Graph (DAG).From list, using flattened list structureįor Directed Acyclic Graph (DAG) implementation, there are 4 main components.BinaryNode, Node with binary tree rules.Sample workflows for tree demonstration!įor Binary Tree implementation, there are 3 main components.īinary Node inherits from Node, so the components in Tree implementation are also available in Binary Tree.Export to dictionary, nested dictionary, or pandas DataFrame.Find single child node based on name, user-defined condition.
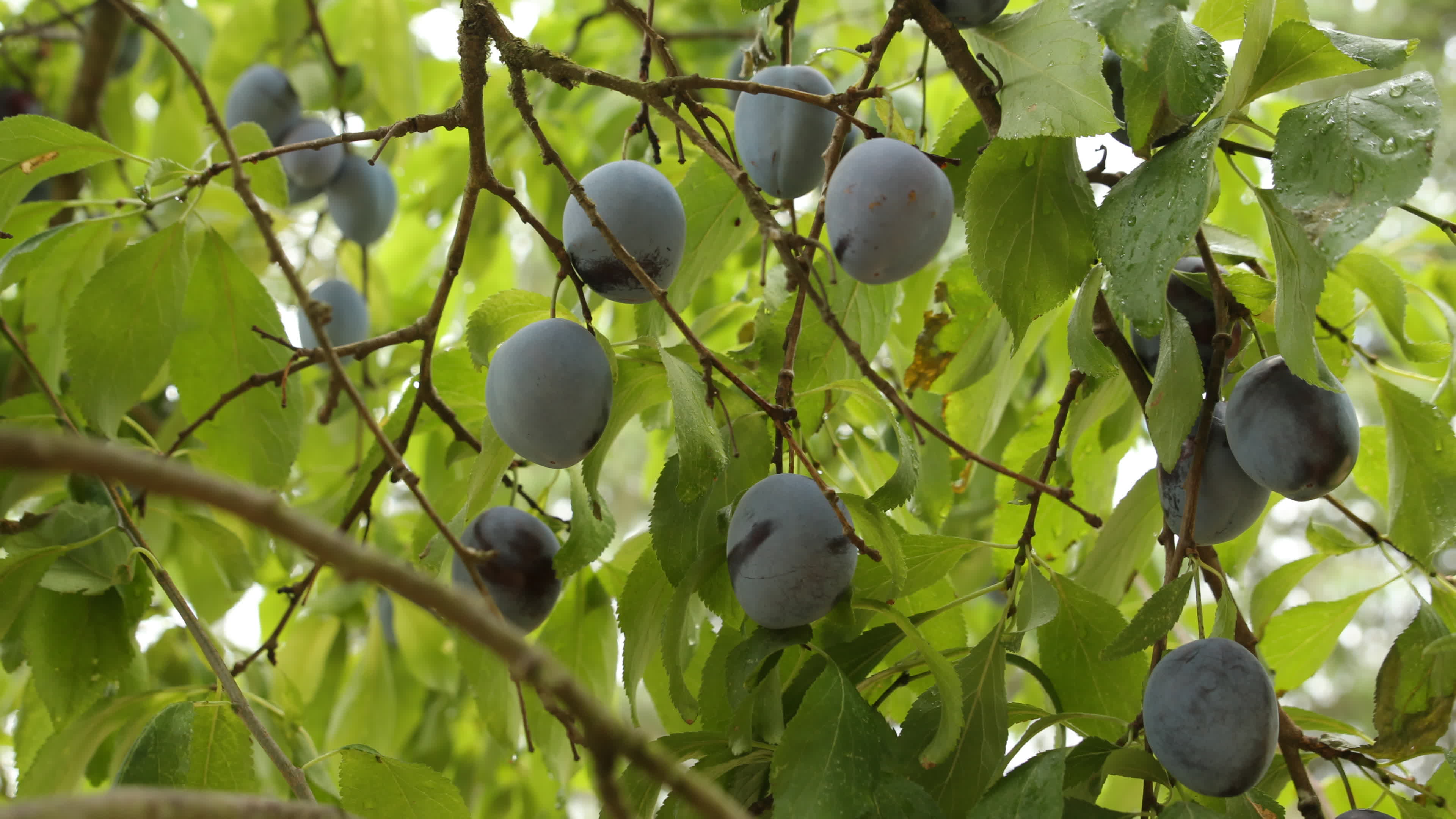
Find multiple child nodes based on user-defined condition.Find single nodes based on name, partial path, full path, attribute value, user-defined condition.Find multiple nodes based on name, partial path, attribute value, user-defined condition.Copy nodes from location to destination.Shift nodes from location to destination.Add only attributes to existing tree using dictionary or pandas DataFrame, using node name.Add nodes and attributes to existing tree using dictionary or pandas DataFrame, using path.Add nodes to existing tree using string.From pandas DataFrame, using paths or parent-child columns.From nested dictionary, using path or recursive structure.From list, using paths or parent-child tuples.Node, BaseNode with node name attribute.There are 3 segments to Big Tree consisting of Tree, Binary Tree, and Directed Acyclic Graph (DAG) implementation.įor Tree implementation, there are 8 main components.
